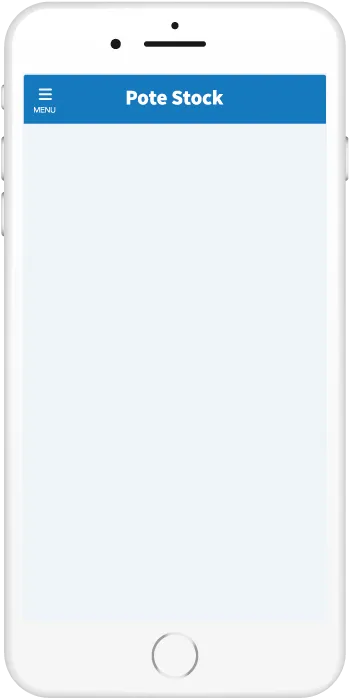
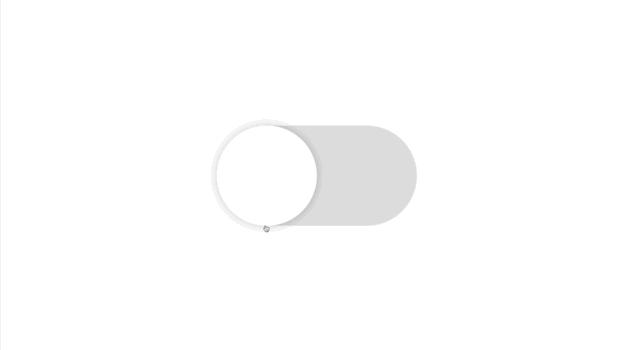
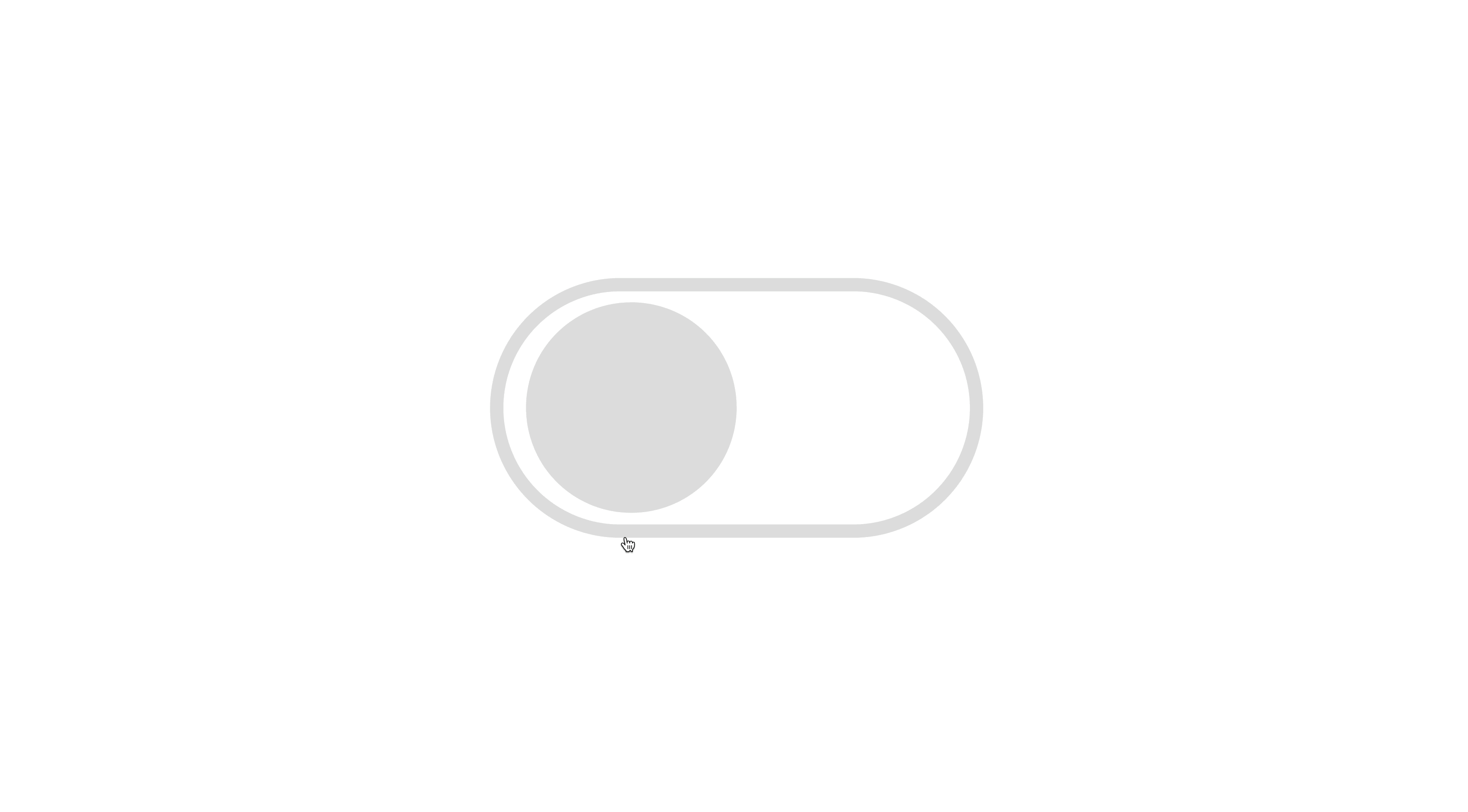
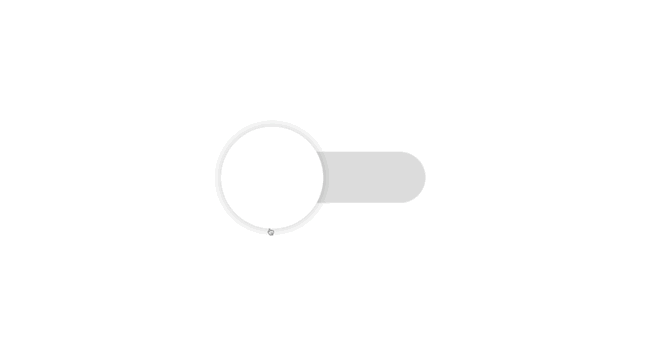
4 toggle button designs made with HTML/CSS
We have put together a design for a toggle button that can switch between two states, ON and OFF, that can be created using only HTML and CSS without requiring Java Script. You can copy and paste anything from things you often see on iOS to things with text.
Simple
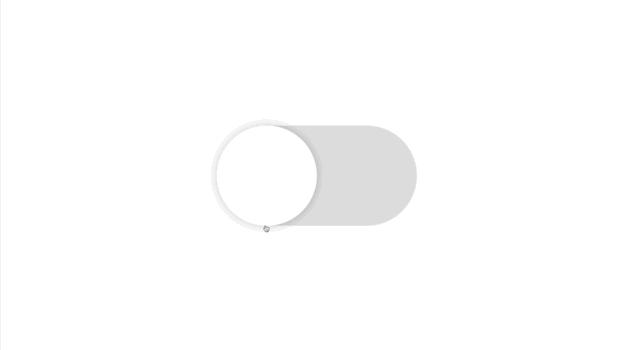
Like iOS
Adjust design
I tried to reproduce the toggle button used in iOS. When placing a label, it is recommended to place it on the left side in consideration of the user's eye movement.
Copy HTML
<label class="toggle-button-1">
<input type="checkbox"/>
</label>
Copy CSS
.toggle-button-1 {
display: inline-block;
position: relative;
width: 100px;
height: 50px;
border-radius: 50px;
background-color: #dddddd;
cursor: pointer;
transition: background-color .4s;
}
.toggle-button-1:has(:checked) {
background-color: #4bd865;
}
.toggle-button-1::after {
position: absolute;
top: 0;
left: 0;
width: 50px;
height: 50px;
border-radius: 50%;
box-shadow: 0 0 5px rgb(0 0 0 / 20%);
background-color: #fff;
content: '';
transition: left .4s;
}
.toggle-button-1:has(:checked)::after {
left: 50px;
}
.toggle-button-1 input {
display: none;
}
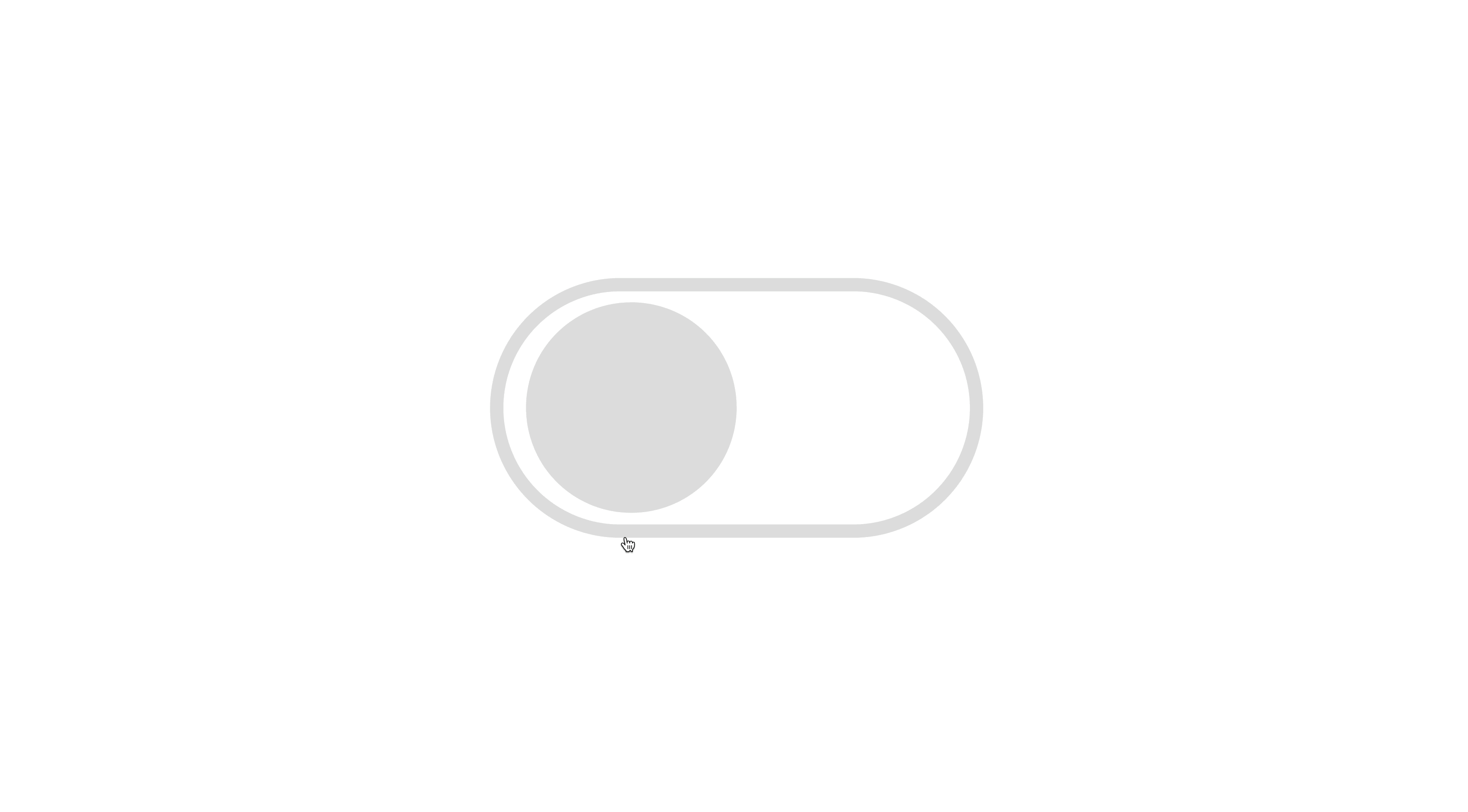
Like iOS(with edges)
Adjust design
Copy HTML
<label class="toggle-button-2">
<input type="checkbox"/>
</label>
Copy CSS
.toggle-button-2 {
display: inline-block;
position: relative;
width: 100px;
height: 50px;
border-radius: 50px;
border: 3px solid #dddddd;
box-sizing: content-box;
cursor: pointer;
transition: border-color .4s;
}
.toggle-button-2:has(:checked) {
border-color: #4bd865;
}
.toggle-button-2::after {
position: absolute;
top: 50%;
left: 5px;
transform: translateY(-50%);
width: 45px;
height: 45px;
border-radius: 50%;
background-color: #dddddd;
content: '';
transition: left .4s;
}
.toggle-button-2:has(:checked)::after {
left: 50px;
background-color: #4bd865;
}
.toggle-button-2 input {
display: none;
}
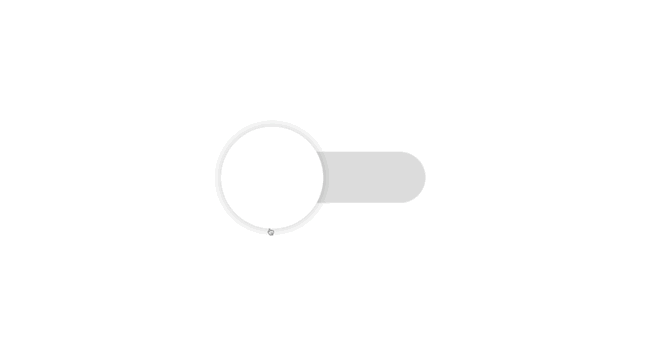
Like iOS(thin background)
Adjust design
Copy HTML
<label class="toggle-button-3">
<input type="checkbox"/>
</label>
Copy CSS
.toggle-button-3 {
display: flex;
align-items: center;
position: relative;
width: 100px;
height: 25px;
margin-top: 12.5px;
border-radius: 50px;
background-color: #dddddd;
cursor: pointer;
transition: background-color .4s;
}
.toggle-button-3:has(:checked) {
background-color: #4bd865;
}
.toggle-button-3::after {
position: absolute;
left: 0;
width: 50px;
height: 50px;
border-radius: 50%;
box-shadow: 0 0 5px rgb(0 0 0 / 20%);
background: #fff;
content: '';
transition: left .4s;
}
.toggle-button-3:has(:checked)::after {
left: 50px;
}
.toggle-button-3 input {
display: none;
}
With text
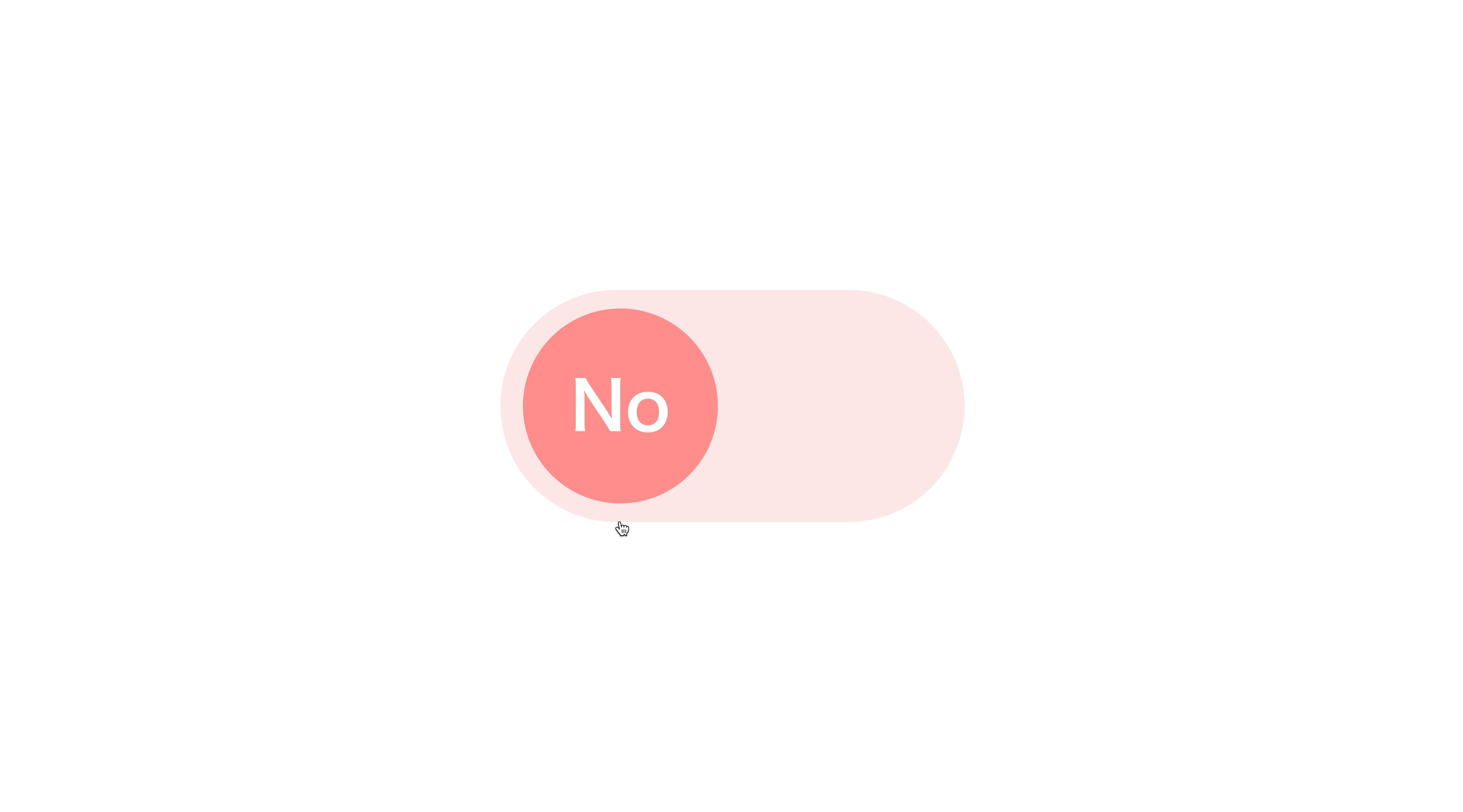
Yes/No
Adjust design
Copy HTML
<label class="toggle-button-4">
<input type="checkbox"/>
</label>
Copy CSS
.toggle-button-4 {
display: flex;
align-items: center;
position: relative;
width: 100px;
height: 50px;
border-radius: 50px;
box-sizing: content-box;
background-color: #ff8d8d33;
cursor: pointer;
transition: background-color .4s;
}
.toggle-button-4:has(:checked) {
background-color: #75bbff33;
}
.toggle-button-4::before {
position: absolute;
left: 5px;
width: 42px;
height: 42px;
border-radius: 50%;
background-color: #ff8d8d;
content: '';
transition: left .4s;
}
.toggle-button-4:has(:checked)::before {
left: 50px;
background-color: #75bbff;
}
.toggle-button-4::after {
position: absolute;
left: 26px;
transform: translateX(-50%);
color: #fff;
font-weight: 600;
font-size: .9em;
content: 'No';
transition: left .4s;
}
.toggle-button-4:has(:checked)::after {
left: 71px;
content: 'Yes';
}
.toggle-button-4 input {
display: none;
}